Build a Gen AI app in minutes with SkySQL, MariaDB Vector, and LlamaIndex
March 14, 2025
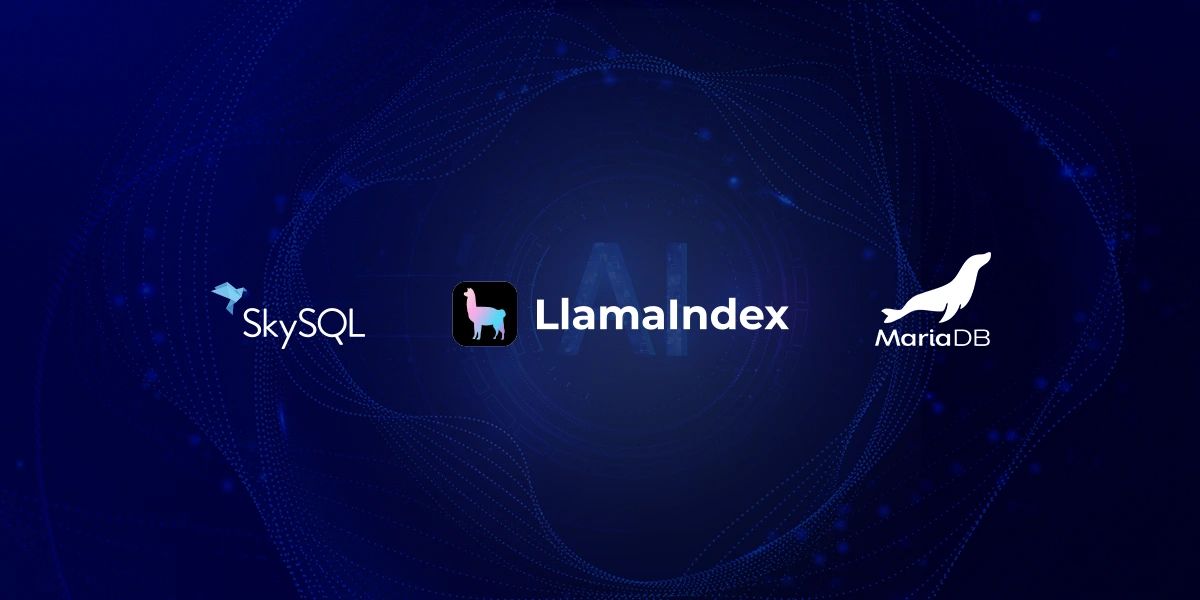
Note: This blog has been updated to reflect the release of MariaDB 11.7, the latest LlamaIndex MariaDB vector store integration, and SkySQL's free serverless developer tier.
MariaDB recently announced full support for vector data in MariaDB 11.7, and SkySQL now integrates MariaDB Vector with LlamaIndex, a leading framework for building generative AI applications. SkySQL introduced a perpetually free serverless database running MariaDB 11.7 to make development even easier, eliminating the need to manually provision, size, or scale your database.
If you're already familiar with vector databases, MariaDB Vector is worth exploring—it’s faster than most alternatives, inherits the transactional and scaling capabilities of a mature open-source database, and eliminates the need to duplicate data into a separate vector store. We’ll dive into the rationale behind these advantages below.
In this blog, we’ll explore the MariaDB features that enable seamless vector support and show how you can use them to build an effective Retrieval-Augmented Generation (RAG) pipeline with LlamaIndex.
Prefer to jump straight into the code? Check out the Colab Notebook here—it takes less than 5 minutes to get started.
Semantic Search with MariaDB Vector
You’re probably familiar with the traditional keyword-based full-text search available in MariaDB/MySQL, where finding results depends on matching exact keywords. But what if you need something more nuanced? Enter vector search. Rather than exact matches, vector stores use similarity to return items that are conceptually related to a query, even if they don’t share identical terms.
Imagine you have a database filled with product reviews, such as: "This laptop is a beast! The M2 chip is incredibly powerful, and the battery life is amazing."
Now, instead of searching for exact words, you can use a vector store to find laptops that received positive sentiment—capturing not just the words but their meaning. This opens up new possibilities for semantic search, recommendation engines, and intelligent discovery in your applications.
Refining Results with LLMs
While the vector store can rapidly surface semantically similar results, the nature of vector searches means the results are approximate—you might still end up with a large set of results that need further refinement. Suppose you want to go deeper: "Show me the top 5 products with negative sentiment and the key reasons for those ratings." Now you need more than just a vector search—you need an LLM capable of generating precise, context-aware answers.
Here’s how it works:
Generate embeddings: Convert your product reviews into high-dimensional vectors, also called embeddings, using models from HuggingFace or OpenAI. Curious about what embeddings are and how they work? You can explore more about the underlying concepts here.
Store in a vector database: Save these embeddings in a vector DB like MariaDB for fast semantic search.
Combine search with LLMs: For user queries, perform a similarity search in the vector store and pass the results as "context" to the LLM. The LLM then generates a refined, targeted response, such as extracting the most relevant sentiments or insights from the reviews.
LlamaIndex simplifies this entire pipeline, orchestrating the process of embedding generation, vector search, and LLM-powered synthesis into one streamlined workflow.
Why MariaDB Vector? It is already raining Vector DBs
Why add complexity by integrating another vector store when you can manage vector embeddings right alongside your existing data? With your data and vector embeddings in MariaDB managed by SkySQL, you get seamless integration—your embeddings are stored, scaled, and secured within the same database as the rest of your data. This approach ensures data consistency, reduces operational overhead, and is often far more cost-effective than relying on external vector databases.
Plus, MariaDB Vector is engineered for performance— recent benchmark testing shows that MariaDB gets ~2X more QPS than pgvector and ~1.5 more than Qdrant. The ANN benchmark results highlight that it is not only fast but efficient with its resource usage. For more details, visit the full performance blog here.
By keeping everything within SkySQL, you gain the advantage of lower latency, auto-scaling, simplified security management, and the confidence that your data and embeddings will always stay in sync.
A peek at the SQL extensions to support Vector operations
Using these vector capabilities is simple but powerful. You define vectors using the VECTOR INDEX
in your CREATE TABLE statement, letting you build indexes that capture the semantic meaning of data.
💡Note - when using frameworks like LlamaIndex, Langchain, etc., you typically don’t need to know any of the SQL extensions below. You just use the higher-level API of the framework. Skip to the next section if this doesn't apply to you.
Here’s how to set it up:
1CREATE TABLE product_reviews (
2 product_name VARCHAR(255),
3 product_id INT,
4 review TEXT,
5 review_embedding BLOB,
6 VECTOR INDEX (review_embedding) -- Define the vector index
7 );
In this example, the review_embedding column stores the semantic meaning of the review column as high-dimensional vectors. These vectors hold much more than just words—they capture the intent, sentiment, and context behind each review.
But how do you generate these embeddings from your text data? Let’s take a practical approach using LlamaIndex with a model from HuggingFace executed locally in Python:
1# Make sure to install the dependences first:
2# pip install llama-index llama-index-embeddings-huggingface
3from llama_index.embeddings.huggingface import HuggingFaceEmbedding
4
5# Load a pre-trained model for text embeddings
6embed_model = HuggingFaceEmbedding(model_name="BAAI/bge-small-en-v1.5")
7embeddings = embed_model.get_text_embedding("This laptop is a beast! ....")
8
9# Insert the embeddings into MariaDB
10cursor = conn.cursor()
11cursor.execute("INSERT INTO product_reviews (product_name, product_id, review, review_embedding) VALUES (%s, %s, %s, Vec_FromText(%s))",
12(product_name, product_id, review, embeddings))
This Python snippet generates a review’s embedding using HuggingFace and seamlessly inserts it into MariaDB. The embedding, now a BLOB, becomes part of your table’s structure, enriching the data with its semantic layer.
Once the embeddings are in place, querying them becomes straightforward with MariaDB’s built-in VEC_Distance function:
1-- Example: Find top 5 products with positive sentiment
2SELECT * FROM product_reviews
3 ORDER BY VEC_Distance(review_embedding, )
4 LIMIT 5;
MariaDB uses the Modified HNSW algorithm for vector searches, defaulting to Euclidean distance for fast and efficient similarity searches. With this, your database is now more than just a collection of rows—it’s a rich, semantically aware system that can surface insights traditional searches might miss.
In this demo, we’ll show how to integrate MariaDB Vector with OpenAI’s LLM using LlamaIndex to build a smart, context-driven AI application.
We begin by launching MariaDB via SkySQL, where you’ll get access to vector capabilities in a cloud environment.
The following snippets highlight key parts of the code, guiding you through how to set up and connect your services. For the full implementation, you can jump into the Google Colab notebook.
1) Launch MariaDB in SkySQL using the API
Alternatively, you can visit app.skysql.com and launch using the portal.
1# Headers for the API requests
2headers = {
3 'Content-Type': 'application/json',
4 'X-API-Key': API_KEY
5}
6
7""" Launch a new SkySQL DB using API """
8# You can change your cloud provider, instance type and storage if you like.
9def launch_skysql_db():
10 url = "https://api.skysql.com/provisioning/v1/services"
11 payload = {
12 "service_type": "transactional",
13 "topology": "es-single",
14 "version": "11.6.0-1-1", # Vector search is available only in this version
15 "provider": "gcp",
16 "region": "us-central1",
17 "architecture": "amd64",
18 "size": "sky-2x8",
19 "storage": 100,
20 "nodes": 1,
21 "name": f"{SKYDB_SERVICE_NAME}",
22 "ssl_enabled": True,
23 "allow_list": [
24 {
25 "comment": "The allowed IP address",
26 "ip": f"{CLIENT_IP}/32"
27 }
28 ]
29 }
30 try:
31 response = requests.post(url, headers=headers, data=json.dumps(payload))
32 response.raise_for_status()
33 return response
34 except requests.exceptions.RequestException as e:
35 print(f"Failed to launch SkySQL DB service: {e}")
36 return None
2) Populate the DB with some ‘Product reviews’
1""" Populate Product reviews in DB """
2
3from llama_index.core.schema import Document
4
5documents = []
6
7def create_skyservice_and_populate():
8 """
9 Creates a schema named 'vector_test' and populates a table named 'product_reviews'
10 with specific laptop reviews.
11 """
12 global documents
13
14 conn = launch_and_get_dbconn() ## Code in colab notebook
15 cursor = conn.cursor()
16
17 try:
18 cursor.execute("CREATE SCHEMA IF NOT EXISTS vector_test")
19 cursor.execute("USE vector_test")
20 cursor.execute("CREATE TABLE IF NOT EXISTS product_reviews" +
21 " (product_name VARCHAR(255), product_id INT, review TEXT)")
22
23 # Specific laptop reviews
24 reviews = [
25 ("Apple MacBook Pro M2", 1, "This thing is a beast! The M2 chip is crazy fast, and the battery lasts forever. The screen is gorgeous, and the keyboard is super comfortable. It's a bit pricey, but totally worth it for the performance and build quality."),
26 ("Dell XPS 13", 2, "I love my XPS 13. It's sleek and powerful, and the screen is amazing. The only downside is the battery life could be a bit better, especially when running demanding tasks."),
27 ("HP Spectre x360", 3, "I'm so glad I got this 2-in-1 laptop. It's perfect for work and play. The design is sleek, and it's really versatile. The battery life could be better, though."),
28 ("Microsoft Surface Laptop Studio", 4, "This is the ultimate laptop for creatives. The touchscreen and stylus make it so easy to work on design projects. It's a bit heavy, but the performance is top-notch."),
29 ("Lenovo ThinkPad X1 Carbon", 5, "I'm a big fan of ThinkPads, and this one doesn't disappoint. It's super durable, the keyboard is amazing, and the battery lasts forever. It's a bit pricey, but it's worth it for the quality."),
30 ("Acer Predator Helios 300", 6, "This is a great gaming laptop for the price. It runs all the latest games smoothly, and the keyboard is comfortable. The only downside is it can get really loud and hot when gaming."),
31 ("Razer Blade 15", 7, "I love the sleek design of this laptop, and it's a powerhouse. The screen is amazing, and the keyboard is perfect for gaming. It's a bit expensive, but it's worth it for the performance."),
32 ("Asus ZenBook 14 OLED", 8, "This laptop is a dream. The OLED screen is stunning, and it's really lightweight. The battery life could be better, but it's still a great laptop."),
33 ("LG Gram", 9, "I was surprised by how light this laptop is! It's perfect for traveling. The performance is decent, but it's not the fastest laptop I've used."),
34 ("Huawei MateBook X Pro", 10, "I love the design of this laptop, but it's a bit hard to find in the US. The screen is great, and the performance is good. The battery life could be better.")
35 ]
36
37 cursor.executemany("INSERT INTO product_reviews " +
38 "(product_name, product_id, review) VALUES (%s, %s, %s)", reviews)
39 conn.commit()
40 print("Schema and table created successfully.")
41
42 cursor.execute("SELECT product_name, product_id, review FROM product_reviews")
43 reviews = [f"Product: {product_name}, ID: {product_id}, Review: {review}" for (product_name, product_id, review) in curso
44
45 ## Convert DB data into LlamaIndex Documents
46 documents = [Document(text=review) for review in reviews]
47 except mysql.connector.Error as err:
48 print(f"Error: {err}")
49
50 finally:
51 ...
3) Populate the MariaDB Vector (Product reviews) using LlamaIndex
Unlike the previous SQL example, you will notice that we now isolate the Vector Index and manage it using our LlamaIndex MariaDB Vector integration.
The code snippet below creates a VectorStoreIndex object in LlamaIndex using our SkySQL managed MariaDB as the actual Vector store.
1""" Create MariaDB Vector store in LlamaIndex """
2
3from llama_index.core import StorageContext, VectorStoreIndex
4from llama_index.core.schema import Document
5from llama_index.vector_stores.mariadb import MariaDBVectorStore
6
7vector_store = MariaDBVectorStore.from_params(
8 database = "vector_test",
9 host = connection_properties["host"],
10 user = connection_properties["user"],
11 password = connection_properties["password"],
12 port = connection_properties["port"],
13) ## Uses the OpenAI number of dimensions (1536) for embeddings and HNSW is the ANN algorithm
14
15storage_context = StorageContext.from_defaults(vector_store=vector_store)
16
17# Creating the index generates embeddings for the documents in the background and saves them
18# into MariaDB with the help of the MariaDBVectorStore
19index = VectorStoreIndex.from_documents(
20 documents, storage_context=storage_context, show_progress=True
21)
4) Time to do semantic searches and engage the LLM
1question = "Show me top 2 laptops that got a positive review along with key reasons cited."
2
3# Using a retriever does a vector search only and returns a list of the top k documents matching semantically the question
4retriever = index.as_retriever(
5 similarity_top_k=3
6)
7retriever_response = retriever.retrieve(question)
8for i, r in enumerate(retriever_response):
9 print(f"\n Vector Store Retriever result {i+1}: {r.text}")
10
11# Now, let's use an LLM on top of the retriever for more meaningful and accurate answers
12
13# Using a query engine does a vector search first and then feeds the results to the LLM
14query_engine = index.as_query_engine()
15response = query_engine.query(question)
16print("\n\nQuery engine response: ", response)
With the MariaDB Vector integration for LlamaIndex, available now in SkySQL, you have a powerful toolkit to build AI applications that leverage cutting-edge semantic search and vector capabilities. By combining the strengths of vector search with LLM-powered insights, you can unlock faster, more meaningful results from your data—all without the usual performance or cost trade-offs. Ready to build smarter, context-driven applications? Dive in and see how easy it is to get started.
Next Steps
Dive deeper into MariaDB Vector to explore its capabilities.
Want to contribute? Help improve the LlamaIndex-MariaDB integration here.
Get started with a free serverless MariaDB cloud database by creating your account at the SkySQL portal.
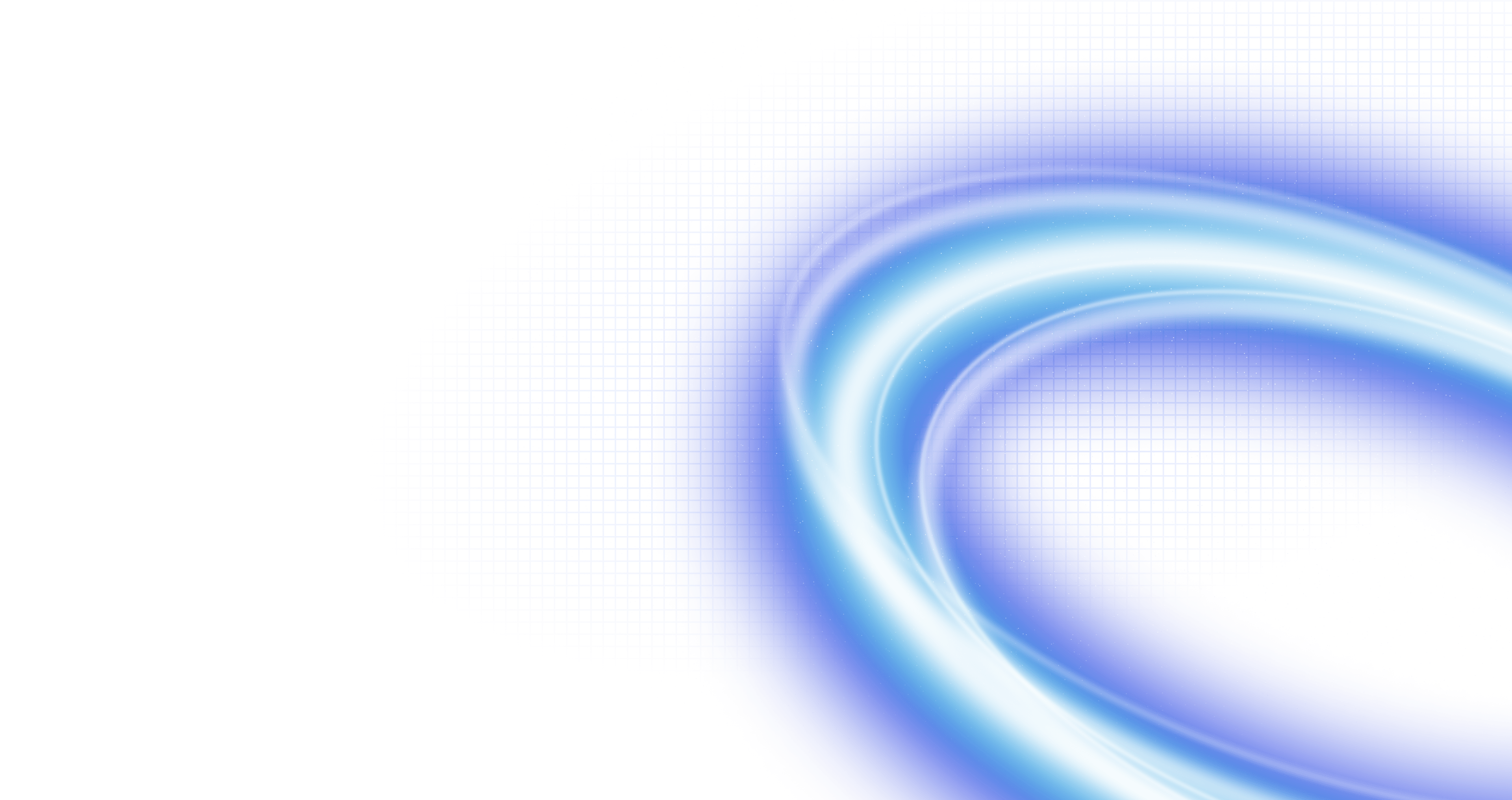
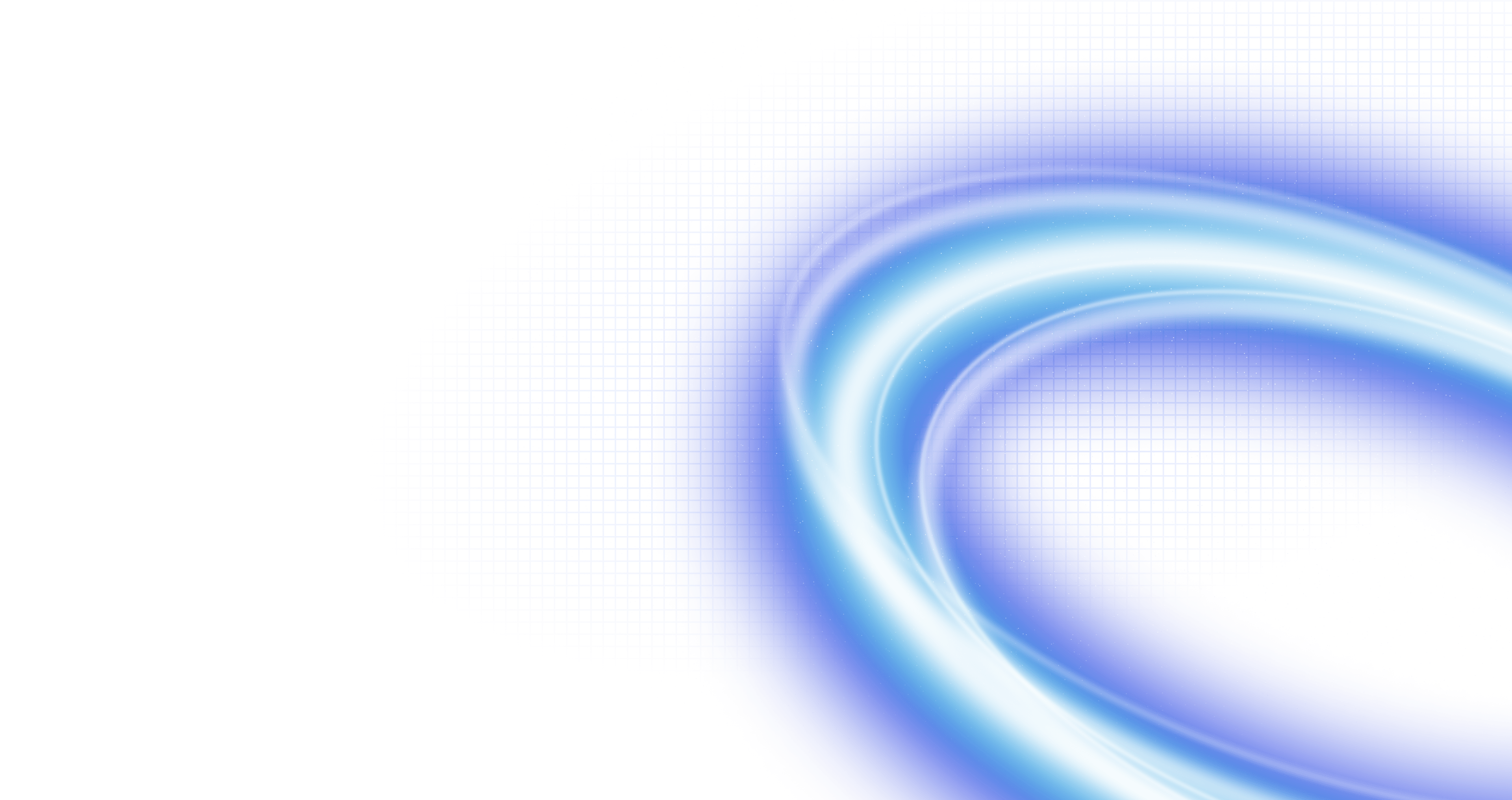